相比上一篇文章所讲的Instrumented Unit Tests,本文所讲的自动化测试Espresso最显著的特点就是,可以与UI相交互。
使用
首先我们在Android Studio中新建一个项目,取名为EspressoTests。同时删除自动生成的一些文件,最终目录结构如下:
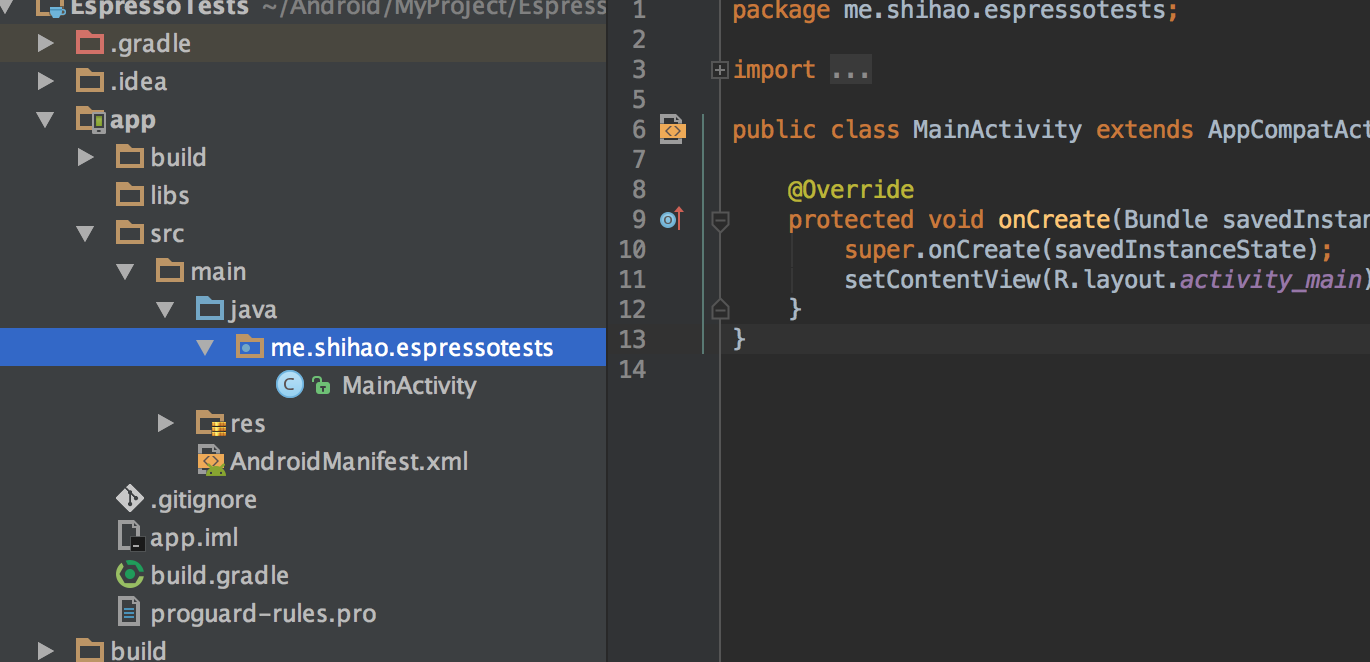
接下来我们看看如何一步一步的使用Espresso,首先在根目录的 build.gradle 文件中添加下面的引入。
build.gradle
Vim
1 2 3 4 5 |
ext { buildToolsVersion = "24.0.1" supportLibVersion = "24.2.0" espressoVersion = "2.2.2" } |
在app目录中的 build.gradle 文件中添加下面的引入,根据提示点击 Sync Now。
build.gradle
Vim
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
dependencies { compile fileTree(dir: 'libs', include: ['*.jar']) // App dependencies compile 'com.android.support:appcompat-v7:' + rootProject.supportLibVersion; compile 'com.android.support:support-annotations:' + rootProject.supportLibVersion; compile 'com.android.support:recyclerview-v7:' + rootProject.supportLibVersion; androidTestCompile 'com.android.support.test.espresso:espresso-core:' + rootProject.espressoVersion; androidTestCompile('com.android.support.test.espresso:espresso-contrib:' + rootProject.espressoVersion) { transitive false }; androidTestCompile 'com.android.support.test.espresso:espresso-web:' + rootProject.espressoVersion; androidTestCompile 'com.android.support:support-annotations:' + rootProject.supportLibVersion; androidTestCompile 'com.android.support:recyclerview-v7:' + rootProject.supportLibVersion; } |
接下来我们编写测试例子,最终的结果如下:
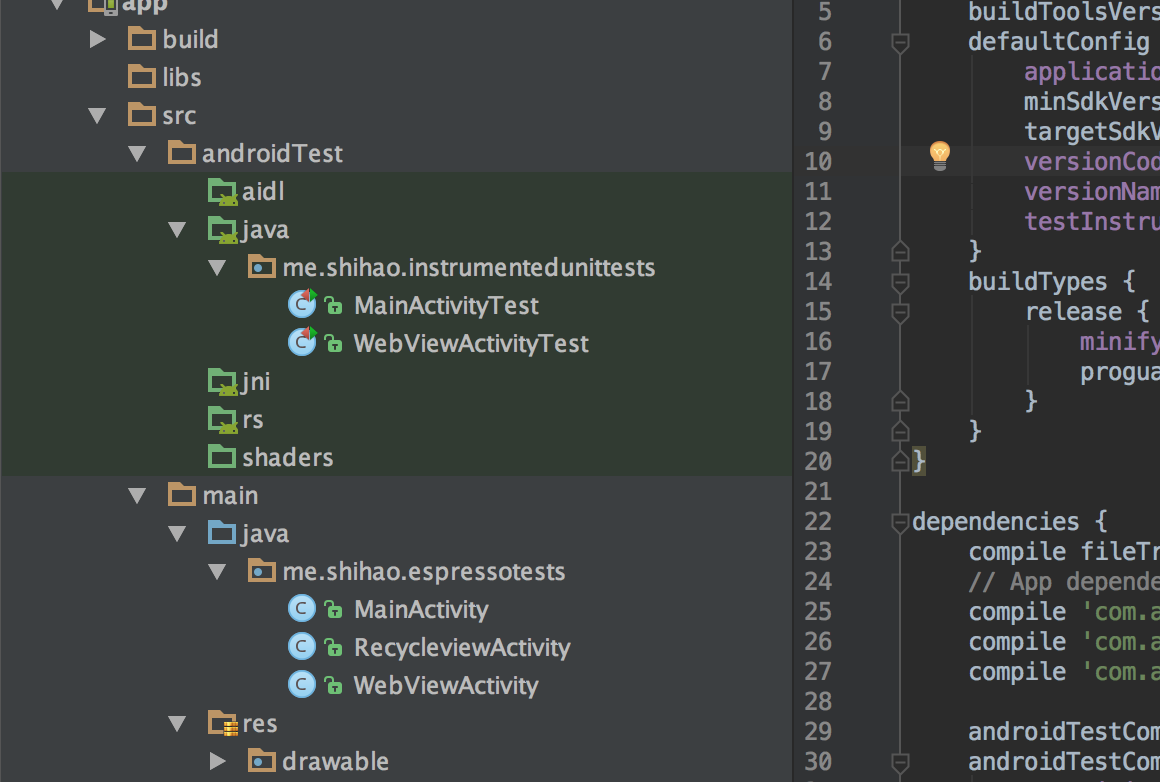
其中MainActivity界面如下,输入框中点击数字后点击计算可以在结果处显示两者相加的值,点击webview跳转到WebViewActivity,点击recycleview跳转到RecycleViewActivity。
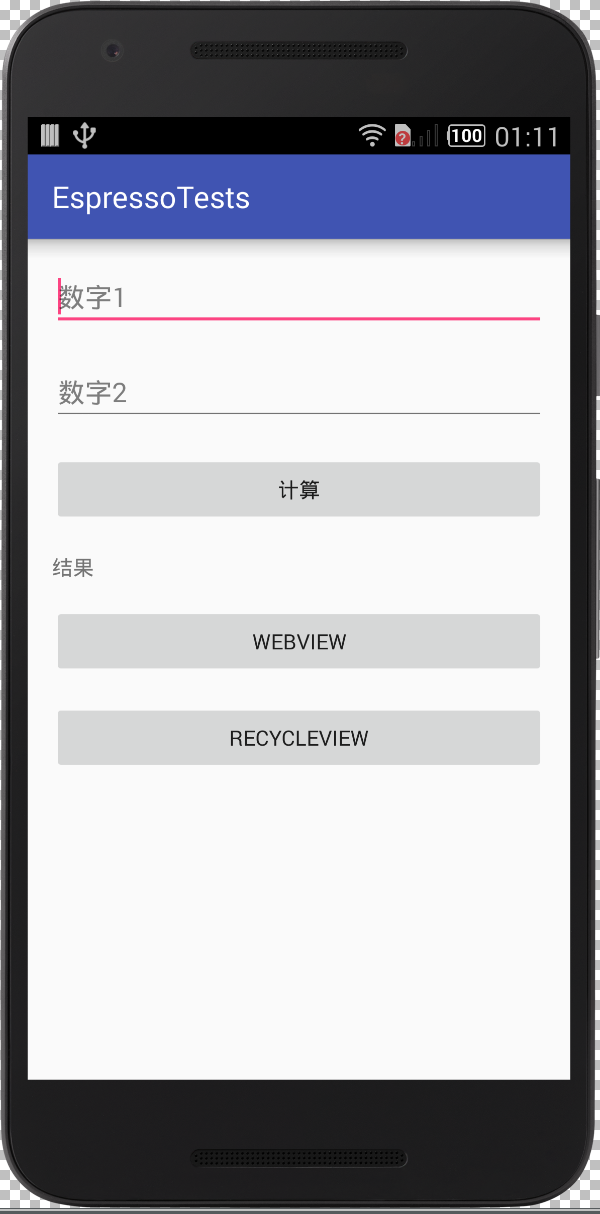
其中WebViewActivity中包含一个webview,可以加载跳转传过来的url。

其中RecycleViewActivity就是一个recycleview的简单使用,实现了一个列表。
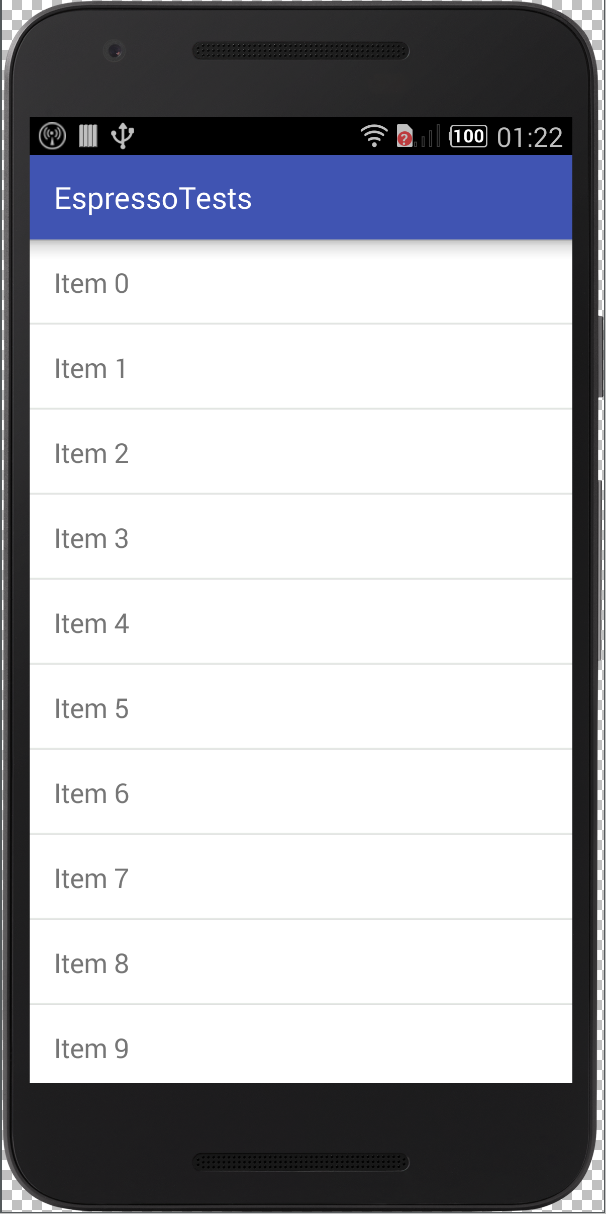
接下来我们看看测试的代码,在代码中又详细的注释。
MainActivityTest.java
Java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 |
package me.shihao.instrumentedunittests; import android.support.test.espresso.Espresso; import android.support.test.espresso.contrib.RecyclerViewActions; import android.support.test.espresso.matcher.ViewMatchers; import android.support.test.espresso.web.assertion.WebViewAssertions; import android.support.test.espresso.web.webdriver.DriverAtoms; import android.support.test.espresso.web.webdriver.Locator; import android.support.test.rule.ActivityTestRule; import android.support.test.runner.AndroidJUnit4; import org.hamcrest.Matchers; import org.junit.Rule; import org.junit.Test; import org.junit.runner.RunWith; import me.shihao.espressotests.MainActivity; import me.shihao.espressotests.R; import static android.support.test.espresso.action.ViewActions.click; import static android.support.test.espresso.action.ViewActions.closeSoftKeyboard; import static android.support.test.espresso.action.ViewActions.typeText; import static android.support.test.espresso.assertion.ViewAssertions.matches; import static android.support.test.espresso.matcher.ViewMatchers.isDisplayed; import static android.support.test.espresso.matcher.ViewMatchers.withId; import static android.support.test.espresso.matcher.ViewMatchers.withText; import static android.support.test.espresso.web.sugar.Web.onWebView; import static android.support.test.espresso.web.webdriver.DriverAtoms.findElement; import static android.support.test.espresso.web.webdriver.DriverAtoms.webClick; /** * Created by shihao on 2017/3/2. */ @RunWith(AndroidJUnit4.class) public class MainActivityTest { @Rule public ActivityTestRule activityTestRule = new ActivityTestRule(MainActivity.class); @Test public void test() { //通过id找到edittext,在里面输入2并关闭输入法 Espresso.onView(withId(R.id.editText)).perform(typeText("2"), closeSoftKeyboard()); //通过id找到edittext,在里面输入5并关闭输入法 Espresso.onView(withId(R.id.editText2)).perform(typeText("5"), closeSoftKeyboard()); //通过id找到button,执行点击事件 Espresso.onView(withId(R.id.button)).perform(click()); //通过id找到textview,并判断是否与文本匹配 Espresso.onView(withId(R.id.textView)).check(matches(withText("计算结果:6"))); Espresso.onView(withId(R.id.textView)).check(matches(withText("计算结果:7"))); } @Test public void testRecycleView() { //通过文本RecycleView找到按钮,并执行点击事件,跳转到RecycleviewActivity Espresso.onView(withText("RecycleView")).perform(click()); //通过文本"Item 0"找到view,并检查是否显示,然后执行点击事件 ,此时会弹出对话框 Espresso.onView(withText("Item 0")).check(matches(isDisplayed())).perform(click()); //通过文本"确定"找到对话框上的确定按钮,执行点击事件,关闭对话框 Espresso.onView(withText("确定")).perform(click()); //通过文本"Item 2"找到view,并检查是否显示,然后执行点击事件,此时会弹出对话框 Espresso.onView(withText("Item 2")).check(matches(isDisplayed())).perform(click()); //执行点击返回按钮事件,关闭对话框 Espresso.pressBack(); //通过id找到recycleview,然后执行滑动事件,滑动到21项 Espresso.onView(ViewMatchers.withId(R.id.recycleview)).perform(RecyclerViewActions.scrollToPosition(21)); //通过文本"Item 20"找到view,并检查是否显示,然后执行点击事件,此时会弹出对话框 Espresso.onView(withText("Item 20")).check(matches(isDisplayed())).perform(click()); //通过文本"确定"找到对话框上的确定按钮,执行点击事件,关闭对话框 Espresso.onView(withText("确定")).perform(click()); //执行点击返回按钮事件,关闭跳转到RecycleviewActivity Espresso.pressBack(); } @Test public void testWebView() { //通过文本RecycleView找到按钮,并执行点击事件,跳转到WebViewActivity Espresso.onView(withText("WebView")).perform(click()); //通过name为"word"找到搜索输入框 onWebView().withElement(findElement(Locator.NAME, "word")) //往输入框中输入字符串"android" .perform(DriverAtoms.webKeys("android")) //通过id为"index-bn"找到"百度一下"按钮 .withElement(DriverAtoms.findElement(Locator.ID, "index-bn")) //执行点击事件 .perform(webClick()) //通过id为"results"找到结果div .withElement(DriverAtoms.findElement(Locator.ID, "results")) //检查div中是否包含字符串"android" .check(WebViewAssertions.webMatches(DriverAtoms.getText(), Matchers.containsString("android"))); //执行点击返回按钮事件,关闭跳转到WebViewActivity Espresso.pressBack(); } } |
MainActivityTest.java
Java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 |
package me.shihao.instrumentedunittests; import android.support.test.espresso.Espresso; import android.support.test.espresso.contrib.RecyclerViewActions; import android.support.test.espresso.matcher.ViewMatchers; import android.support.test.espresso.web.assertion.WebViewAssertions; import android.support.test.espresso.web.webdriver.DriverAtoms; import android.support.test.espresso.web.webdriver.Locator; import android.support.test.rule.ActivityTestRule; import android.support.test.runner.AndroidJUnit4; import org.hamcrest.Matchers; import org.junit.Rule; import org.junit.Test; import org.junit.runner.RunWith; import me.shihao.espressotests.MainActivity; import me.shihao.espressotests.R; import static android.support.test.espresso.action.ViewActions.click; import static android.support.test.espresso.action.ViewActions.closeSoftKeyboard; import static android.support.test.espresso.action.ViewActions.typeText; import static android.support.test.espresso.assertion.ViewAssertions.matches; import static android.support.test.espresso.matcher.ViewMatchers.isDisplayed; import static android.support.test.espresso.matcher.ViewMatchers.withId; import static android.support.test.espresso.matcher.ViewMatchers.withText; import static android.support.test.espresso.web.sugar.Web.onWebView; import static android.support.test.espresso.web.webdriver.DriverAtoms.findElement; import static android.support.test.espresso.web.webdriver.DriverAtoms.webClick; /** * Created by shihao on 2017/3/2. */ @RunWith(AndroidJUnit4.class) public class MainActivityTest { @Rule public ActivityTestRule activityTestRule = new ActivityTestRule(MainActivity.class); @Test public void test() { //通过id找到edittext,在里面输入2并关闭输入法 Espresso.onView(withId(R.id.editText)).perform(typeText("2"), closeSoftKeyboard()); //通过id找到edittext,在里面输入5并关闭输入法 Espresso.onView(withId(R.id.editText2)).perform(typeText("5"), closeSoftKeyboard()); //通过id找到button,执行点击事件 Espresso.onView(withId(R.id.button)).perform(click()); //通过id找到textview,并判断是否与文本匹配 Espresso.onView(withId(R.id.textView)).check(matches(withText("计算结果:6"))); Espresso.onView(withId(R.id.textView)).check(matches(withText("计算结果:7"))); } @Test public void testRecycleView() { //通过文本RecycleView找到按钮,并执行点击事件,跳转到RecycleviewActivity Espresso.onView(withText("RecycleView")).perform(click()); //通过文本"Item 0"找到view,并检查是否显示,然后执行点击事件 ,此时会弹出对话框 Espresso.onView(withText("Item 0")).check(matches(isDisplayed())).perform(click()); //通过文本"确定"找到对话框上的确定按钮,执行点击事件,关闭对话框 Espresso.onView(withText("确定")).perform(click()); //通过文本"Item 2"找到view,并检查是否显示,然后执行点击事件,此时会弹出对话框 Espresso.onView(withText("Item 2")).check(matches(isDisplayed())).perform(click()); //执行点击返回按钮事件,关闭对话框 Espresso.pressBack(); //通过id找到recycleview,然后执行滑动事件,滑动到21项 Espresso.onView(ViewMatchers.withId(R.id.recycleview)).perform(RecyclerViewActions.scrollToPosition(21)); //通过文本"Item 20"找到view,并检查是否显示,然后执行点击事件,此时会弹出对话框 Espresso.onView(withText("Item 20")).check(matches(isDisplayed())).perform(click()); //通过文本"确定"找到对话框上的确定按钮,执行点击事件,关闭对话框 Espresso.onView(withText("确定")).perform(click()); //执行点击返回按钮事件,关闭跳转到RecycleviewActivity Espresso.pressBack(); } @Test public void testWebView() { //通过文本RecycleView找到按钮,并执行点击事件,跳转到WebViewActivity Espresso.onView(withText("WebView")).perform(click()); //通过name为"word"找到搜索输入框 onWebView().withElement(findElement(Locator.NAME, "word")) //往输入框中输入字符串"android" .perform(DriverAtoms.webKeys("android")) //通过id为"index-bn"找到"百度一下"按钮 .withElement(DriverAtoms.findElement(Locator.ID, "index-bn")) //执行点击事件 .perform(webClick()) //通过id为"results"找到结果div .withElement(DriverAtoms.findElement(Locator.ID, "results")) //检查div中是否包含字符串"android" .check(WebViewAssertions.webMatches(DriverAtoms.getText(), Matchers.containsString("android"))); //执行点击返回按钮事件,关闭跳转到WebViewActivity Espresso.pressBack(); } } |
WebViewActivityTest.java
Java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 |
package me.shihao.instrumentedunittests; import android.content.Intent; import android.support.test.espresso.web.action.AtomAction; import android.support.test.espresso.web.assertion.WebViewAssertions; import android.support.test.espresso.web.sugar.Web; import android.support.test.espresso.web.webdriver.DriverAtoms; import android.support.test.espresso.web.webdriver.Locator; import android.support.test.rule.ActivityTestRule; import android.support.test.runner.AndroidJUnit4; import org.hamcrest.Matchers; import org.junit.Rule; import org.junit.Test; import org.junit.runner.RunWith; import me.shihao.espressotests.WebViewActivity; import static android.support.test.espresso.web.sugar.Web.onWebView; import static android.support.test.espresso.web.webdriver.DriverAtoms.findElement; import static android.support.test.espresso.web.webdriver.DriverAtoms.webClick; import static org.hamcrest.Matchers.containsString; /** * Created by shihao on 2017/3/3. */ @RunWith(AndroidJUnit4.class) public class WebViewActivityTest { @Rule public ActivityTestRule activityTestRule = new ActivityTestRule(WebViewActivity.class, false); @Test public void test() { //传递数据到WebViewActivity Intent intent = new Intent(); intent.putExtra(WebViewActivity.EXTRA_URL, "http://m.baidu.com"); activityTestRule.launchActivity(intent); //通过name为"word"找到搜索输入框 onWebView().withElement(findElement(Locator.NAME, "word")) //往输入框中输入字符串"android" .perform(DriverAtoms.webKeys("android")) //通过id为"index-bn"找到"百度一下"按钮 .withElement(DriverAtoms.findElement(Locator.ID, "index-bn")) //执行点击事件 .perform(webClick()) //通过id为"results"找到结果div .withElement(DriverAtoms.findElement(Locator.ID, "results")) //检查div中是否包含字符串"android" .check(WebViewAssertions.webMatches(DriverAtoms.getText(), Matchers.containsString("android"))); } } |
接下来我们运行测试一下MainActivityTest,看看效果如何

测试结果与我们预期的一样,有一个不匹配。手机运行效果如下:
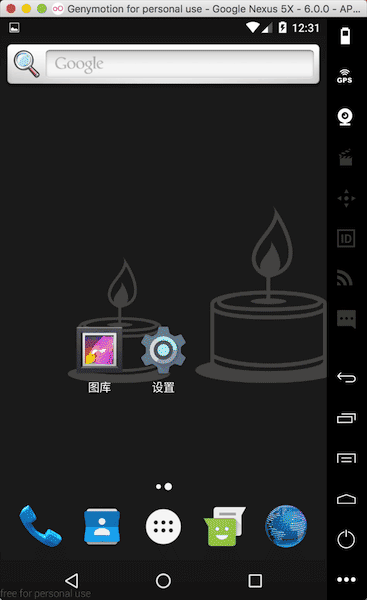
接下来我们就看一看如何在测试中与多个应用界面相交互,欢迎查看下一篇文章:
Demo代码已经放到了Github上:https://github.com/fodroid/EspressoTests